Most of the time I have too many ideas for apps and which ones to build first, the App Idea Evaluator lets you create a list of ideas and rank them against a number of criteria. It also provides a few overview graphs to sum up the overall picture of your ideas.
http://bit.ly/appideaevaluator
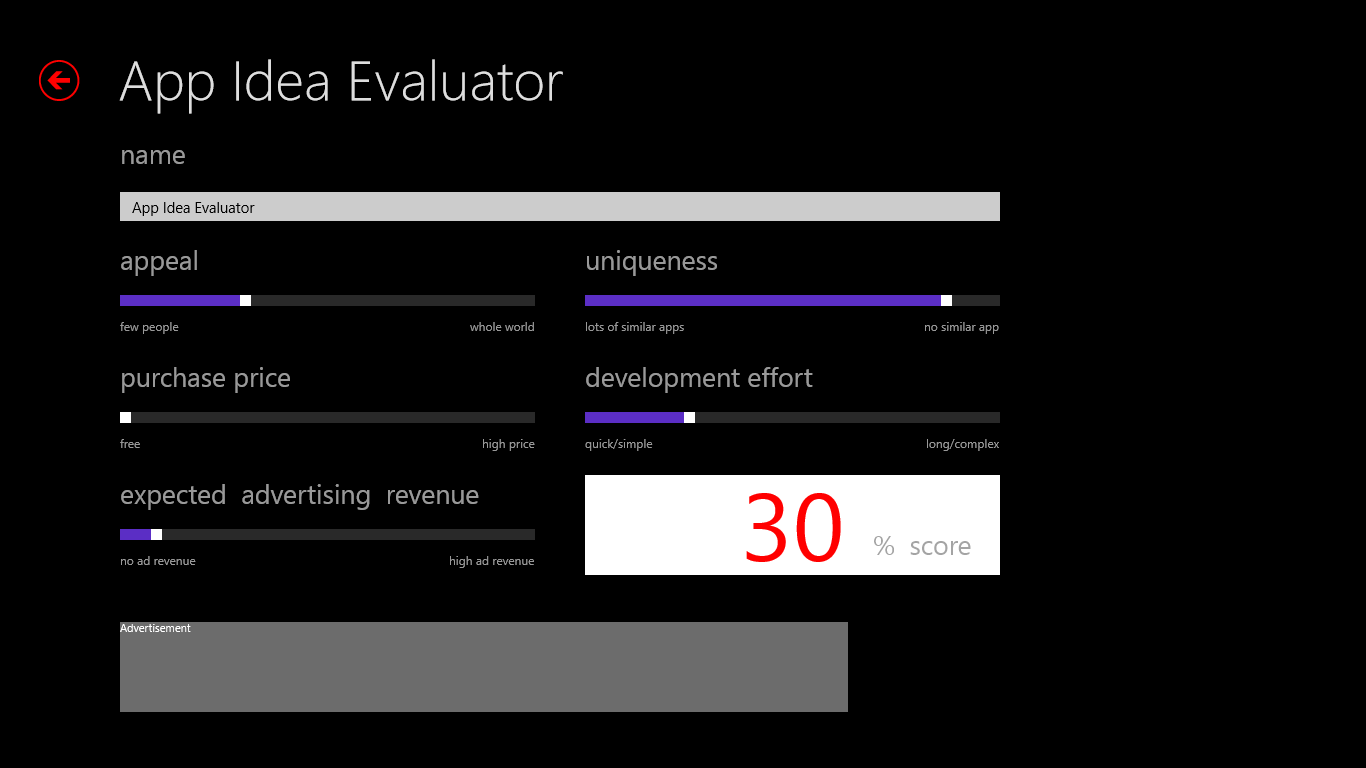
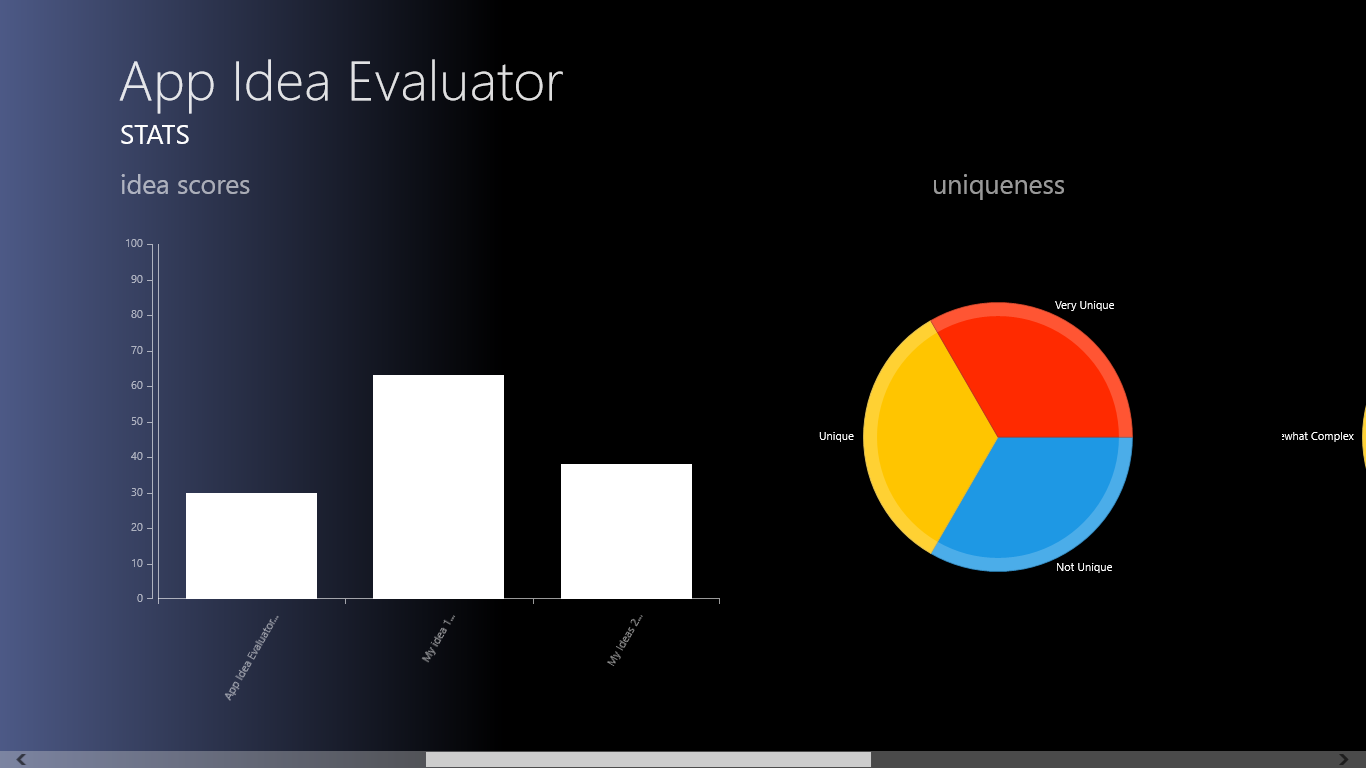
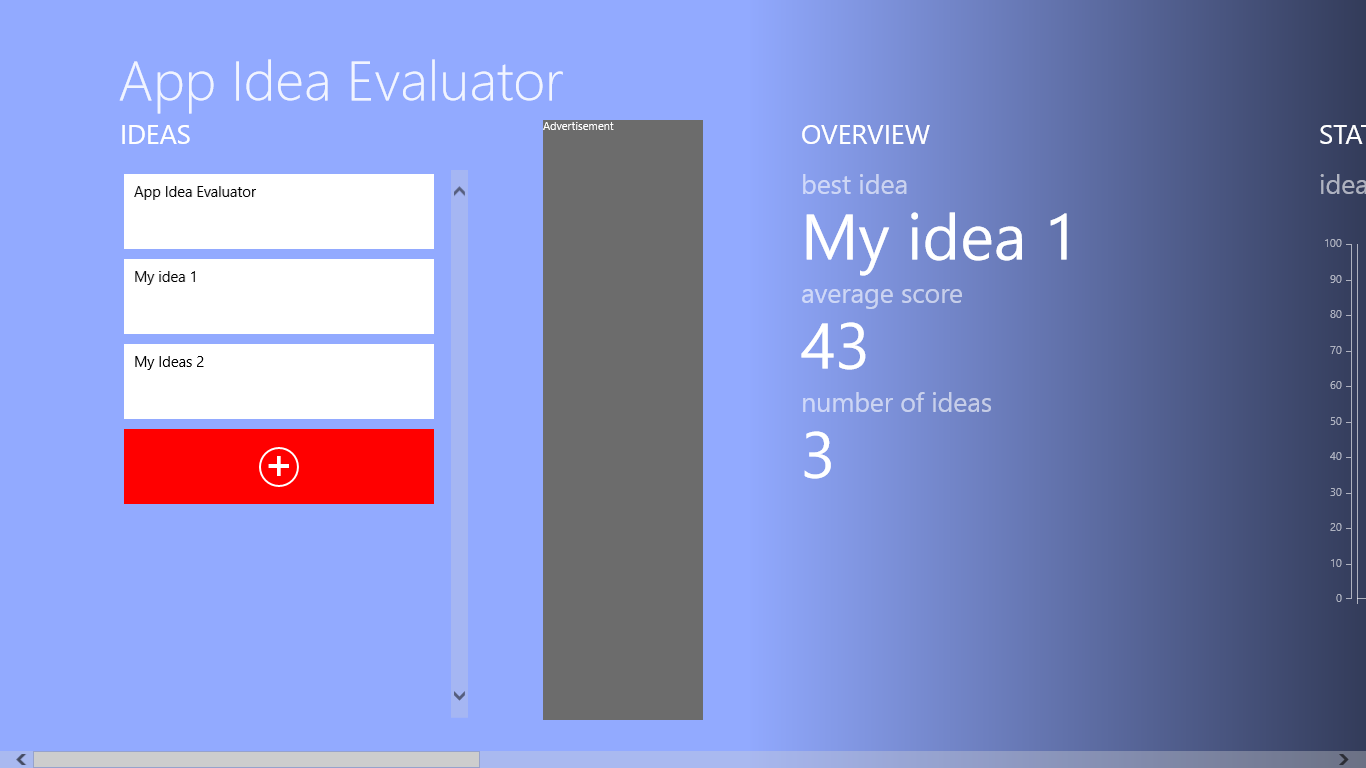
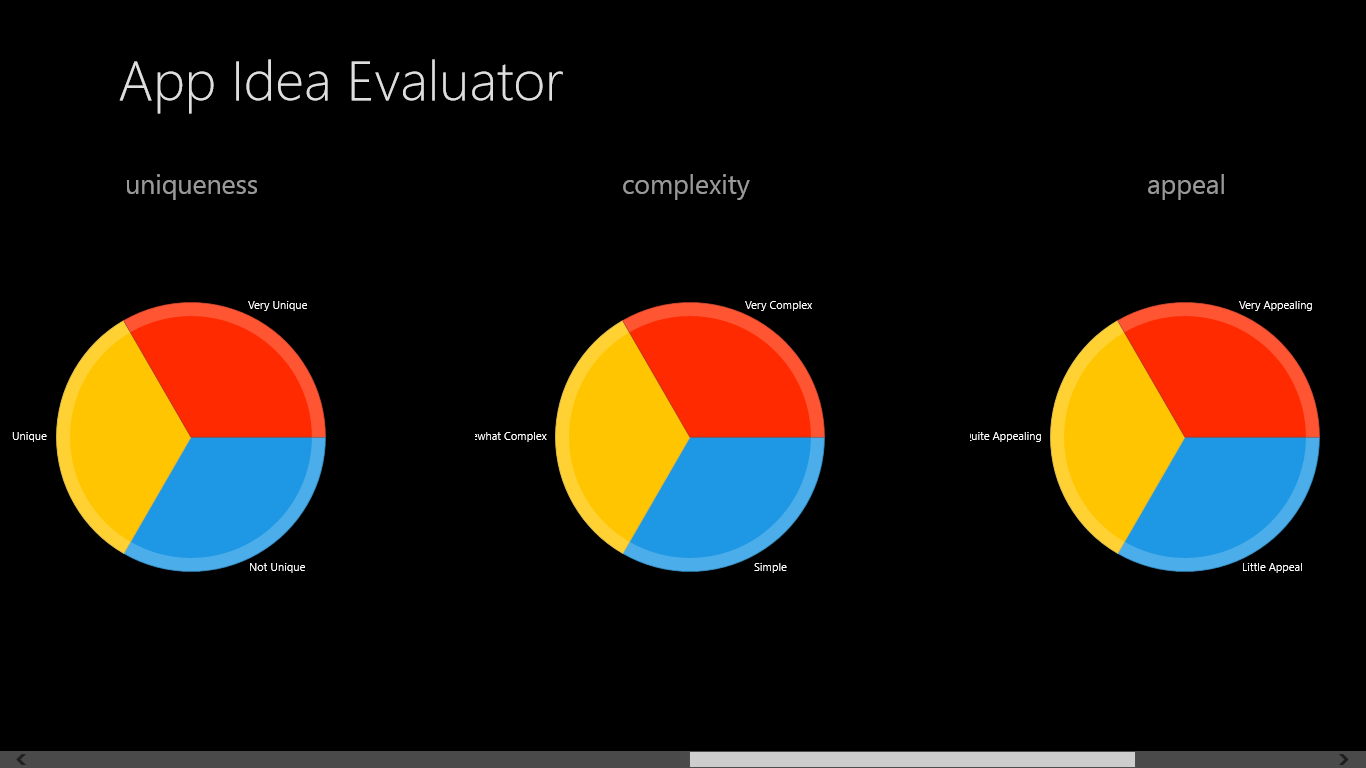
SHARE:
A common UI pattern is to have a list of things on screen and at the end of the list have a plus icon (or something) that triggers your “add new item” code.
For example, in the Weather app:
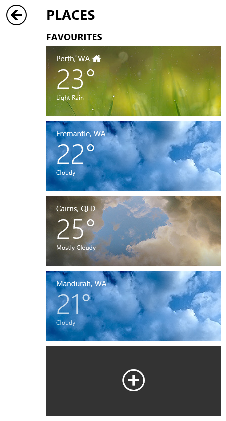
For example, say we want to display a list of friends in a XAML ListView and at the bottom of the list have a “+” that adds a new friend.
public class Friend
{
public string Name { get; set; }
}
The first step is to enable us to differentiate between what is an actual friend in the list and what is the “add new placeholder” item.
More...
SHARE:
(The below is an extract from the “Time Management and Motivation” chapter of my book Keeping Software Soft)
The Pomodoro Technique is a simple time management system developed by Francesco Cirillo.
A "Pomodoro" is an indivisible, all-or-nothing unit of time that is exactly 25 minutes long. A Pomodoro is ether completed or not, for example there is no concept of a completing a half-Pomodoro.
The basics of the technique are:
- Work for a 25 minutes (one Pomodoro)
- Record the completion of a Pomodoro
- Take a 5 minute rest break
- Repeat
- After every 4th Pomodoro, take a longer rest break of between 15-30 minutes
The splitting up of time into 25 minute chunks helps to focus the mind on what small tasks(s) can be accomplished next. If a Pomodoro is horribly interrupted to the point where it is considered void, then a short break can be taken and a new one started. The recording of every completed Pomodoro contributes to the feeling of a sense of progress and can further enhance motivation and energy. During the 5 minute rest break the mind should be allowed to rest; the current task should not be thought about but rather: go for walk, get a drink, stretch, gaze out of the window, etc.
On top of this basic idea, the technique also suggests recording the number of interruptions that occur during a Pomodoro.
Interruptions are classified as either:
- Internal: things that pop into a person's head that are unrelated to the current task, e.g. "what shall I have for lunch today"
- External: things that occur from outside influences such as phone calls, instant message notifications, etc.
By recording the types and number of interruptions, the data can be used to reduce or eliminate them over time. This can involve turning off telephones, email, and instant messaging during a Pomodoro and having dedicated Pomodoro(s) to responding to emails, etc.
The technique, when applied correctly can result in less tiredness while programming and an overall increase in productivity. There are some programmers who do not like the forced "interruption" of a rest break every 25 minutes and feel that it interrupts their concentration. It is a technique that should be trialled by each individual for a reasonable period of time to see if it can be of benefit.
There are many electronic Pomodo timers available for a whole host of platforms to help time the 25 minutes.
For more detail about the technique, see the official website.
SHARE:
I had a nice comment from @mbartosovsky on Twitter about women in IT and thought I would create a few charts that represent my 12+ years experience in software development.
The results are from me thinking back so the numbers may be slightly inaccurate, however the overall picture is I feel (unfortunately) correct.
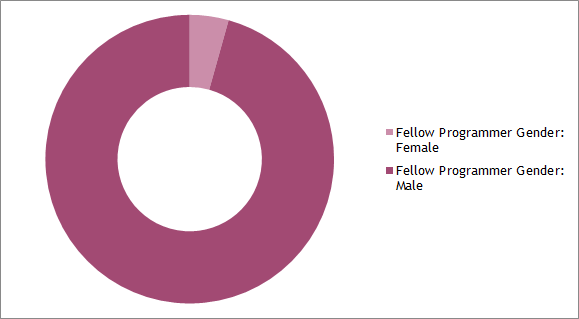
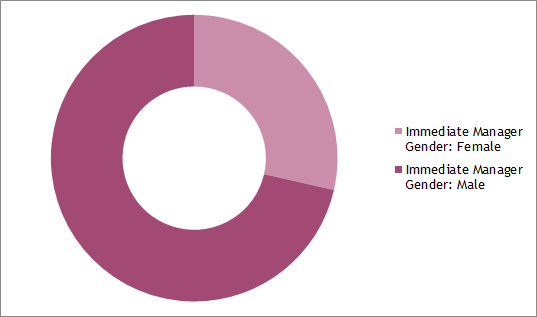
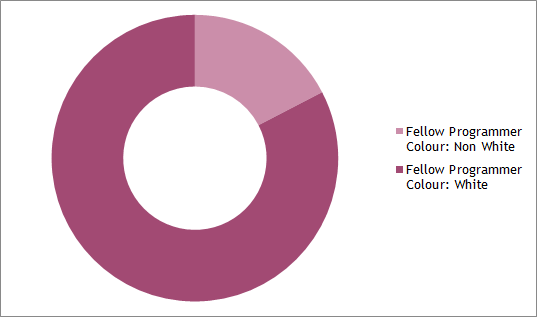
SHARE:
The below is an excerpt from the latest chapter “An Introduction to Unit Testing With xUnit.net” from my book Keeping Software Soft.
xUnit.net provides a number of ways for checking (asserting) results are as expected.
The following explanatory tests shown the different types of assertions that xUnit.net supports:
using System;
using System.Collections.Generic;
using Xunit;
namespace KeepingSoftwareSoftSamples.XUnitTestingDemo
{
public class XUnitAssertExamples
{
[Fact]
public void SimpleAssertsThatOneValueEqualsAnother()
{
Assert.Equal(1, 2); // fail
Assert.Equal("hello", "hello"); // pass
Assert.NotEqual(1, 2); // pass
Assert.NotEqual("hello", "hello"); // fail
}
[Fact]
public void BooleanAsserts()
{
Assert.True(true); // pass
Assert.True(false); // fail
Assert.False(false); // pass
Assert.False(true); // fail
// Don't do this
Assert.True(1 == 1); // pass
}
[Fact]
public void Ranges()
{
const int value = 22;
Assert.InRange(value, 21, 100); // pass
Assert.InRange(value, 22, 100); // pass
Assert.NotInRange(value, 999, 99999); // pass
Assert.InRange(value, 23, 100); // fail
}
[Fact]
public void Nulls()
{
Assert.Null(null); // pass
Assert.NotNull("hello"); // pass
Assert.NotNull(null); // fail
}
[Fact]
public void ReferenceEquality()
{
var objectA = new Object();
var objectB = new Object();
Assert.Same(objectA, objectB); // fail
Assert.NotSame(objectA, objectB); // pass
}
[Fact]
public void AnIEnumberableContainsASpecificItem()
{
var days = new List<string>
{
"Monday",
"Tuesday"
};
Assert.Contains("Monday", days); // pass
Assert.Contains("Friday", days); // fail
Assert.DoesNotContain("Friday", days); // pass
}
[Fact]
public void IEnumerableEmptiness()
{
var aCollection = new List<string>();
Assert.Empty(aCollection); // pass
aCollection.Add("now no longer empty");
Assert.NotEmpty(aCollection); // pass
Assert.Empty(aCollection); // fail
}
[Fact]
public void IsASpecificType()
{
Assert.IsType<string>("hello"); // pass
Assert.IsNotType<int>("hello"); // pass
Assert.IsType<int>("hello"); // fail
}
[Fact]
public void IsAssignableFrom()
{
const string stringVariable = "42";
Assert.IsAssignableFrom<string>(stringVariable); // pass
Assert.IsAssignableFrom<int>(stringVariable); // fail
}
}
}
To learn more about xUnit.net check out my xUnit.net Pluralsight course.
You can start watching with a Pluralsight free trial.


SHARE:
I was asked a question about this on Twitter, so thought I’d create a quick post about it.
The example below shows how to use a DataTemplate that is defined in XAML with a ListView control that is dynamically created in the code behind.
1 Create a New WPF Application
Create WPF application project in Visual Studio.
2 Create The basic XAML
Replace the contents of the MainWindow XAML to the following:
<Window x:Class="WpfApplication1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Window.Resources>
<DataTemplate x:Key="DaysDataTemplate">
<Grid Background="Cyan">
<TextBlock Text="{Binding}"></TextBlock>
</Grid>
</DataTemplate>
</Window.Resources>
<Grid Name="RootContainer" />
</Window>
This defines a DataTemplate called DaysDataTemplate that simply binds the value and has a cyan background so we can see if it is being applied.
We also give the root container (Grid) a name RootContainer so we can reference it later in the code behind.
More...
SHARE:
Today I released the first iteration of my first ever book via Leanpub.com.
To use the word iteration with the word book seems odd, traditionally books are written in their entirety, go through some reviews and editors and eventually get published into the world. Then at some point some errata is released on the publishers website, followed sometime later by a second edition.
Lean publishing turns this on its head and says that the book can be written bit-by-bit with the readers able to contribute by providing feedback about what they like and don’t like so far and what they’d like to see in a final version. This is great as the author(s) can make a book that better suits the people that will be reading it. Readers that buy an in-progress version get free updates as the book progresses.
My book is called Keeping Software Soft, it is based on the premise that software should be easy to change. It’s aimed primarily at apprentice and journeyman software developers and contains my distilled experiences (so far...) after 12+ years of commercial software development. The book provides practical guidance on how to keep software as soft as we can.
It’s currently about 20% complete, and you can buy it today and get free updates as new chapters are released.
Find out more about the Lean Publishing Manifesto here.
SHARE:
Until xUnit officially supports* Windows store apps you can get xUnit working with your WinRT app by doing the following:
In Visual Studio 2012, go to Tools menu, Extensions and Updates; search for and install “xUnit.net runner for Visual Studio 2012”.

In Visual Studio 2012, go to Tools menu, Extensions and Updates; search for and install “xUnit Test Library Template”.

You may have to restart Visual Studio…
More...
SHARE:
I just released an update to my open source feature toggling library FeatureToggle.
Version 1.2.0 add support for using feature toggles in your Windows 8 WinRT .Net (C#) app. For this initial release, the NuGet package for Windows8 app installs the source code, rather than a binary reference. This is so it will always build to whatever architecture you are compiling against (Any CPU, x86, x64, ARM).
This release also provides a XAML value converter (FeatureToggleToVisibilityConverter) to make binding the visibility of UI element to a feature toggle easier.
What To Use Feature Toggles For
Typically feature toggles (sometimes called feature flags, flippers, etc.) enable you to keep one branch of source code and deploy code to production with “half done” stuff. This half done stuff is (typically) toggled at the UI level so it is not available to users.
With Windows 8 in-app-purchases (IAPs), you could also use the FeatureToggle library as a unified way of enabling those extra IAP features that a user has paid for.
Getting Started with FeatureToggle in a Windows 8 App
Install FeatureToggle NuGet Package
Create a new Visual C# Windows Store project (Blank App XAML is fine for this demo).
Install the FeatureToggle NuGet package by either:
- Right0click project, Manage NuGet Packages, search for FeatureToggle, click the Install button; or
- In the Package Manager Console window, type “Install-Package FeatureToggle” and hit enter.
You will notice two folders added to the project: “FeatureToggleCode” (which you don’t need to change) and “FeatureToggleSample” which contains an example toggle.
Create a Feature Toggle
Create a new class called “AwesomeFeature” that looks like this:
internal class AwesomeFeature : JasonRoberts.FeatureToggle.AlwaysOffFeatureToggle
{
}
More...
SHARE:
[Writing this on the train home so may be a bit rushed – sorry :) ]
Install the Bits
- In Visual Studio 2012, go to Tools menu, Extensions and updates.
- Search for “SQLite for Windows Runtime” – install it.
- In your Windows Store app, install the NuGet package called “sqlite-net” – these are the LINQy wrappers over SQLite.
Use SQLite
Create an “entity” that you want to store in a table, e.g. Customer.
Create some plain properties, choose one to be your primary key, e.g.
[SQLite.PrimaryKey, SQLite.AutoIncrement]
public int Id { get; set; }
public string Name { get; set; }
Create a constant to be the filename of the SQLite db on disk:
private const string dbFileName = "data.dat";
More...
SHARE: