I was asked a question about this on Twitter, so thought I’d create a quick post about it.
The example below shows how to use a DataTemplate that is defined in XAML with a ListView control that is dynamically created in the code behind.
1 Create a New WPF Application
Create WPF application project in Visual Studio.
2 Create The basic XAML
Replace the contents of the MainWindow XAML to the following:
<Window x:Class="WpfApplication1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Window.Resources>
<DataTemplate x:Key="DaysDataTemplate">
<Grid Background="Cyan">
<TextBlock Text="{Binding}"></TextBlock>
</Grid>
</DataTemplate>
</Window.Resources>
<Grid Name="RootContainer" />
</Window>
This defines a DataTemplate called DaysDataTemplate that simply binds the value and has a cyan background so we can see if it is being applied.
We also give the root container (Grid) a name RootContainer so we can reference it later in the code behind.
3 Dynamically Add A Control in Code
In the code behind of MainWindow, modify the code too look like this:
using System.Windows;
using System.Windows.Controls;
namespace WpfApplication1
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
// Create new dynamic control that we want to add
var daysList = new ListView();
// use the DataTemplate that is defined in the XAML of this window
daysList.ItemTemplate = (DataTemplate) this.Resources["DaysDataTemplate"];
// If the DataTemplate was defined elsewhere, e.g. in the App.xaml :
//daysList.ItemTemplate = (DataTemplate)Application.Current.Resources["DaysDataTemplate"];
// Create some sample data for the list
daysList.ItemsSource = new[] {"Monday", "Tuesday", "etc."};
// Dynamically add the new list control to the root container
RootContainer.Children.Add(daysList);
}
}
}
4 Run the Application
Run the application and you should see the list with cyan background:
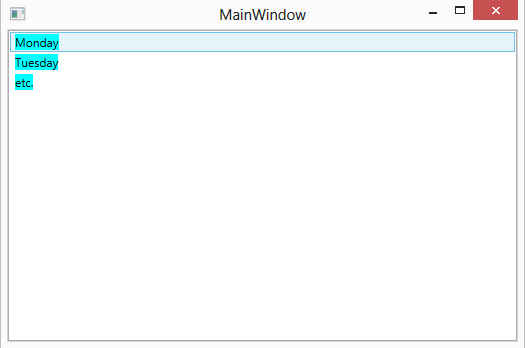
SHARE: