Utilizing the dynamic features of C# and the Dynamic Language Runtime (DLR), among other things, allows C# programs to interoperate with other dynamic languages built on top of the DLR.
For example, Python has the ability to chain operators, for example the expression “10 < 15 < 20” returns true: 10 is less than 15 and 15 is less than 20.
To use a chained Python expression from C# code, IronPython can be used.
To get started (in this example a C# console application) first install the IronPython NuGet package. This allows the application to interoperate with Python code.
The following code shows a console application that evaluates a user-entered number “n” against the Python expression “10 < n < 20”:
using IronPython.Hosting;
using Microsoft.Scripting;
using Microsoft.Scripting.Hosting;
using static System.Console;
namespace PythonCSharp
{
class Program
{
static void Main(string[] args)
{
const string pythonExpression = "10 < n < 20";
int n;
while (true)
{
WriteLine($"Enter a value for n where {pythonExpression}");
n = int.Parse(ReadLine()); // cast error check omitted
ScriptEngine pythonEngine = Python.CreateEngine();
ScriptScope pythonScope = pythonEngine.CreateScope();
// set the value of the Python token "n" in the expression 10 < n < 20
pythonScope.SetVariable("n", n);
ScriptSource pythonScriptSource = pythonEngine.CreateScriptSourceFromString(pythonExpression, SourceCodeKind.Expression);
// Execute the Python expression and get the return value
dynamic dynamicResult = pythonScriptSource.Execute(pythonScope);
WriteLine($"{pythonScriptSource.GetCode().Replace("n", n.ToString())} = {dynamicResult}");
WriteLine();
}
}
}
}
Running the console application allows the user to enter a value for “n” and evaluate it against the Python expression as the following image shows:
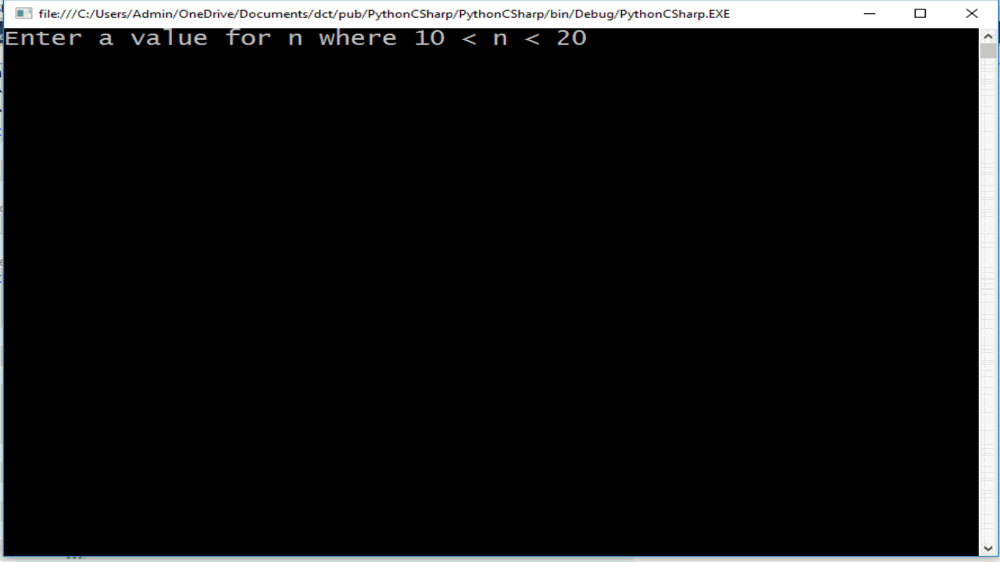
To learn more about how C# makes dynamic possible, some potential use cases, and more detailed on implementing custom types check out my Dynamic C# Fundamentals Pluralsight course.
You can start watching with a Pluralsight free trial.


SHARE: