In addition to doing some fun/weird/useful/annoying things in Console applications, we can also set foreground and background colours.
To set colours we use the Console.BackgroundColor and Console.ForegroundColor properties.
When we set these properties we supply a ConsoleColor. To reset the colours back to the defaults we can call the ResetColor() method.
using System;
namespace ConsoleColorDemo
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Default initial color");
Console.BackgroundColor = ConsoleColor.White;
Console.ForegroundColor = ConsoleColor.Black;
Console.WriteLine("Black on white");
Console.WriteLine("Still black on white");
Console.ResetColor();
Console.WriteLine("Now back to defaults");
Console.ReadLine();
}
}
}
The above code produces the following output:
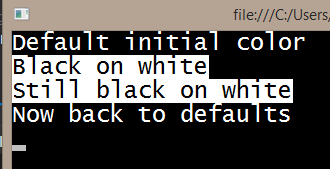
We can enumerate the available Console colours. The following code prints all the colours:
var allColors = Enum.GetValues(typeof (ConsoleColor));
foreach (var c in allColors)
{
Console.ForegroundColor = (ConsoleColor)c;
Console.WriteLine(c.ToString());
}
This produces the following output:
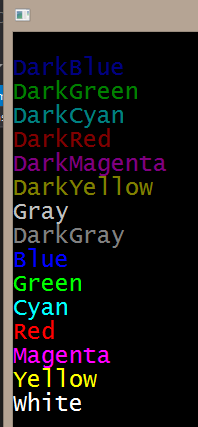
For more console related tips, check out my Building .NET Console Applications in C# Pluralsight course.
You can start watching with a Pluralsight free trial.


SHARE: