Azure Functions allow the creation of Serverless event driven applications with minimal effort. We can create Azure Functions using C# (or other languages) right inside the Azure Web app.
Functions sit inside a function app and each function can be configured to be executed in response to a number of triggers such as a BlobTrigger, EventHubTrigger, etc. One of the triggers is the Timer trigger that can be configured to execute the function on a specified schedule.
To get get started, once you’ve created an Azure account and logged in, head to http://functions.azure.com/ and this will allow a quickstart experience to develop your function (you may be asked to create a new Function App as a container for your functions so go ahead and follow the prompts to do this).
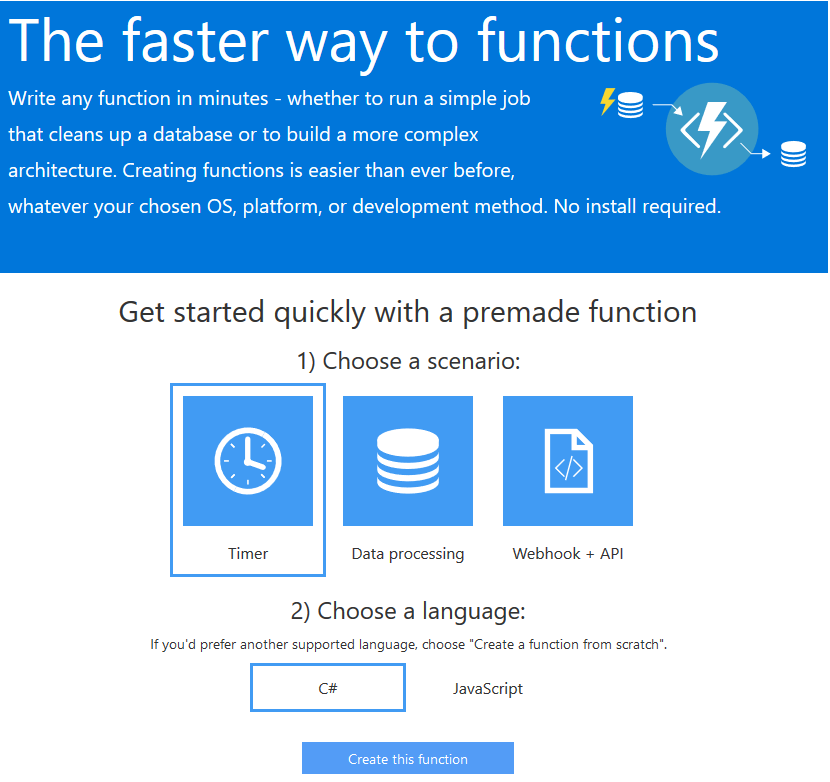
Click “Create this function” and you’ll be taken to the editor.
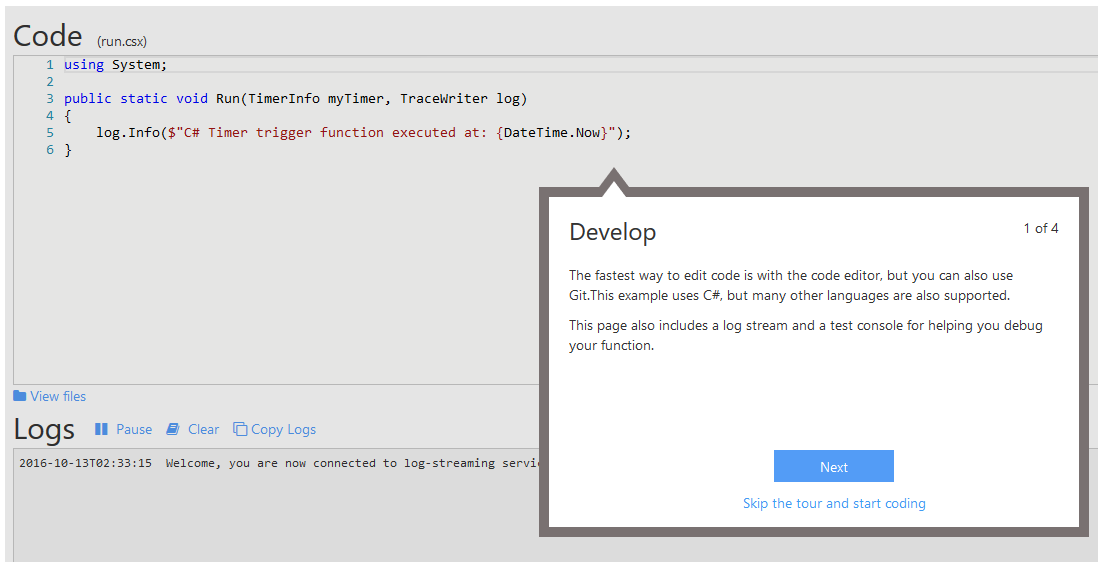
In the code section (within the Run method) we can write code that is executed when the function executes.
Clicking on the Integrate tab allows the specification of the schedule in CRON format.

To have the function execute every five minutes we would specify: 0 */5 * * * *
A function can have Outputs, one of which is a Twilio output. At the time of writing this is in an early beta/experimental phase and I couldn’t get it to work correctly. However because we can write C#, we can send an SMS by using the Twilio REST API. You need to sign up for a Twilio trial account and this will give you a Twilio AccountSID and an authorisation token.
Click the Develop tab and add the following code (using your Twilio SID and auth token):
using System;
using Twilio;
public static void Run(TimerInfo myTimer, TraceWriter log)
{
log.Info($"C# Timer trigger function executed at: {DateTime.Now}");
string accountSid = "YOUR SID HERE";
string authToken = "YOUR TOKEN HERE";
var client = new TwilioRestClient(accountSid, authToken);
client.SendMessage(
"+614xxxxxxxx", // Insert your Twilio from SMS number here
"+614xxxxxxxx", // Insert your verified (trial) to SMS number here
"hello from Azure Functions!" + DateTime.Now
);
}
(+614 is the dialling code for Australian mobiles so you should replace this with whatever makes sense for the Twilio account phone numbers you’ve created.)
Click Save and you’ll get some compilation errors because we haven’t yet added the Twilio NuGet package to be used by our code.
Click the View Files link under the code editing window and add a new file (by clicking the plus icon). Add a file called project.json with the following content:
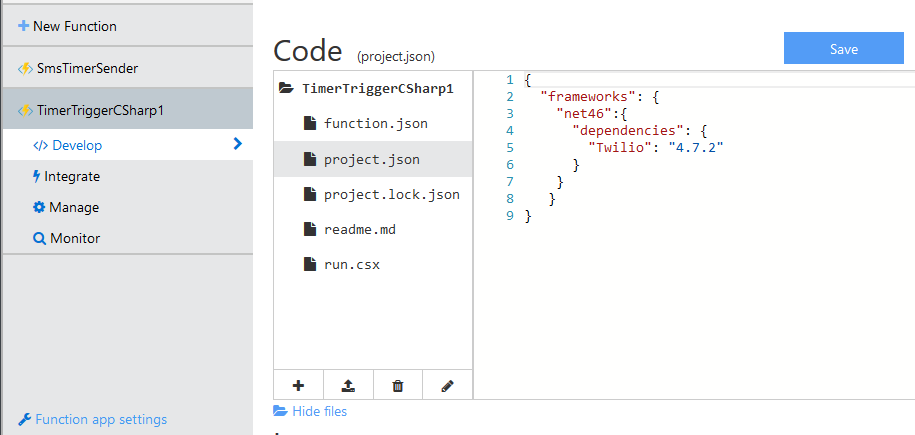
Click Save and in the logs section you should see the package installed:
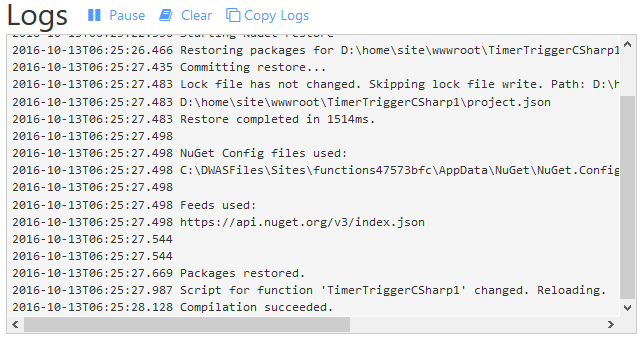
Now every five minutes you’ll get a text message sent!
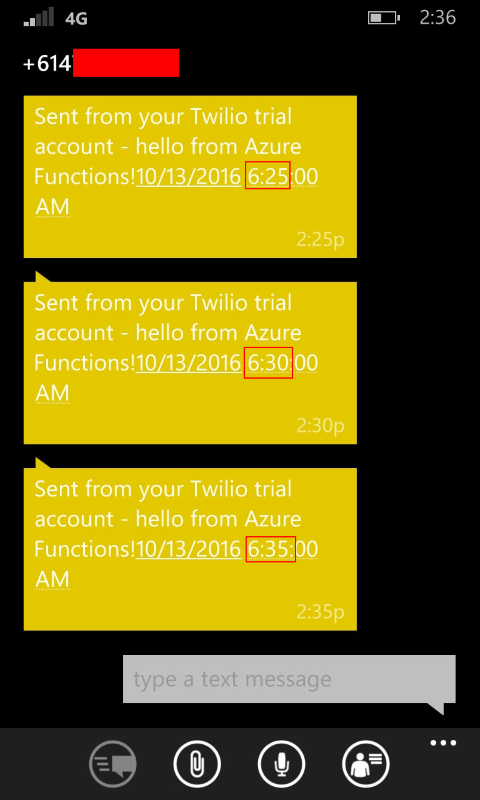
Just remember to disable/delete the function or you will continue to get messages sent. You can do this by clicking on the Manage tab and choosing the appropriate option:

SHARE: