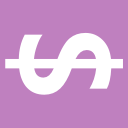
I just released version 2 of a new open source project called InAppPurchaseToggle.
It simplifies querying if the current user has paid for a particular in-app-purchase (IAP).
It’s convention based and allows creation of strongly-typed objects to represent each IAP offer that you’ve configured in the win8 dev dashboard for your app.
How To Use (updated for v2.0.0)
Create a new Windows Store app project (C#/XAML)in Visual Studio.
Install the NuGet package InAppPurchaseToggle.
Currently the package just installs the source in a folder called “InAppPurchaseToggleCode”, this is to simply multi platform (ARM, x86, x64) until NuGet is able to provide dll versions for different platforms.
Single In App Offer
Assuming you had set up an in app offer in the developer dashboard for your app called “AwesomeFeature1” you would create the following class:
internal class AwesomeFeature1 : InAppPurchaseToggle.SinglePurchaseToggleBase {}
There’s no need to implement anything else. Note: the name of the class exactly matches the name of the offer that was created in the developer dashboard.
To test of the current user has purchased AwesomeFeature1 you’d write the following code:
var f = new AwesomeFeature1();
if (f.IsPurchased)
{
// do stuff to enable feature
}
By default InAppPurchaseToggle will use the real Windows store licence data, for testing purposes you can manually set one of 3 simulated / debugging versions:
- SimulatedAlwaysPurchasedStoreGateway - always returns true for .IsPurchased
- SimulatedNeverPurchasedStoreGateway - always returns false for .IsPurchased
- CurrentAppSimulatorStoreGateway – uses the WinRT CurrentAppSimulator.LicenseInformation (see msdn for more info on how to configure this)
To use one of these 3 options, set the WindowsStoreGateway property after creating an instance of AwesomeFeature1:
f.StoreGateway = new SimulatedAlwaysPurchasedStoreGateway();
f.StoreGateway = new SimulatedNeverPurchasedStoreGateway();
f.StoreGateway = new CurrentAppSimulatorStoreGateway();
Note: you’d probably surround this with a conditional compiler directive so as to ensure your release version doesn’t accidentally ship with a simulated version:
var f = new AwesomeFeature1();
#if DEBUG
f.StoreGateway = new SimulatedAlwaysPurchasedStoreGateway();
#endif
if (f.IsPurchased)
{
// do stuff to enable feature
}
Repeat In App Offer
A repeat in app offer is a way of allowing multiple purchased.
For example a slot machine app where you can buy 100 coins. In the developer dashboard you would could create multiple in app offers, e.g. Coin_1, Coin_2 … Coin_100
In the app, you’d create a toggle and derive from RepeatPurchaseToggleBase:
public class Coin : InAppPurchaseToggle.RepeatPurchaseToggleBase
{
protected override int SetAvailableStoreInstances()
{
return 100;
}
}
In the overridden method SetAvailableStoreInstances just return the total number of repeats as configured in the dev dashboard.
This Coin can now be used in the following ways:
var t = new Coin();
int totalInstancesAvailable = t.AvailableStoreInstances;
int nextUnpurchased = t.GetNextLowestUnpurchasedInstance();
int totalInstancesPurchasedSoFar = t.GetTotalPurchased();
bool userPurchasedAllInstances = t.IsAllPurchased();
bool isThirtySecondInstancePurchased = t.IsInstancePurchased(32);
By default the naming scheme used is toggle name (the name of the derived type, in this case Coin) followed by an underscore followed by the instance number. To use the non underscore version:
public class Coin : InAppPurchaseToggle.RepeatPurchaseToggleBase
{
protected override int SetAvailableStoreInstances()
{
return 100;
}
protected override InAppPurchaseToggle.IRepeatPurchaseToggleNameInstanceFormatter SetNameInstanceFormatter()
{
return new InAppPurchaseToggle.NameNumberFormatter();
}
}
If you want to use a completely different scheme, create your own version of IRepeatPurchaseToggleNameInstanceFormatter and return an instance of it in SetNameInstanceFormatter method.
SHARE: