In Silverlight 4 we can present HTML content to the user.
The WebBrowser Control
For example to display the content of DontCodeTired.com we can set the Source property in XAML:
<WebBrowser Name="webBrowser" Source="http://www.dontcodetired.com" />
Or we can use the Navigate method of WebBrowser:
webBrowser.Navigate(new Uri(@"http://www.dontcodetired.com"));
The user can navigate to links, right-click and open in new tab (which opens up tab in a full web browser), view source, etc.
To use the WebBrowser the Silverlight app must be running out of browser (OOB), if you view when embedded in a web page (i.e. not OOB) the control displays a message saying that it can only be used in Out-of-Browser mode.
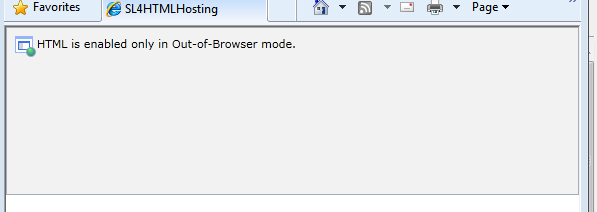
WebBrowserBrush
Using the new WebBrowserBrush (previously HtmlBrush in beta 4) we can use the contents of a WebBrowser to fill another element.
For example, to fill an Ellipse with the content of a WebBrowser named "webBrowser" we define a WebBrowserBrush and sets its SourceName:
<Ellipse>
<Ellipse.Fill>
<WebBrowserBrush SourceName="webBrowser" />
</Ellipse.Fill>
</Ellipse>
The following example creates an ellipse which is filled with a blurred version of the content of a WebBrowser. One thing to note is that we hook into the WebBrowser's LoadCompleted event to ensure the ellipse is updated whenever a page has been loaded into the WebBrowser. When run it looks like the image below:
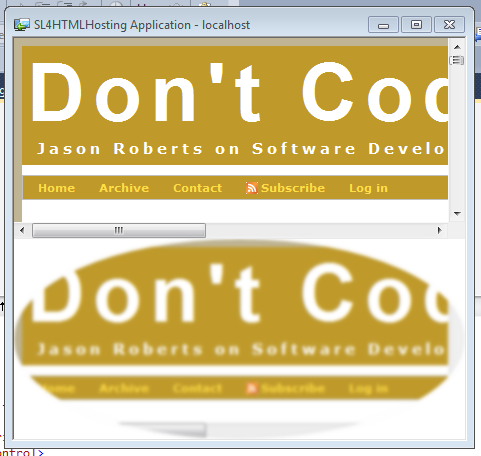
XAML:
<UserControl x:Class="SL4HTMLHosting.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="400">
<Grid x:Name="LayoutRoot" Background="White">
<Grid.RowDefinitions>
<RowDefinition Height="1*" />
<RowDefinition Height="1*" />
</Grid.RowDefinitions>
<WebBrowser Name="webBrowser"
Source="http://www.dontcodetired.com"
Grid.Row="0"
LoadCompleted="webBrowser_LoadCompleted">
</WebBrowser>
<Ellipse HorizontalAlignment="Stretch"
VerticalAlignment="Stretch"
Grid.Row="1">
<Ellipse.Effect>
<BlurEffect Radius="5" />
</Ellipse.Effect>
<Ellipse.Fill>
<WebBrowserBrush x:Name="browserBrush"
SourceName="webBrowser" />
</Ellipse.Fill>
</Ellipse>
</Grid>
</UserControl>
Code Behind:
using System.Windows.Controls;
namespace SL4HTMLHosting
{
public partial class MainPage : UserControl
{
public MainPage()
{
InitializeComponent();
}
private void webBrowser_LoadCompleted(object sender, System.Windows.Navigation.NavigationEventArgs e)
{
browserBrush.Redraw();
}
}
}
Interacting With WebBrowser Content
The following example uses WebBrowser.NavigateToString which allows a string of HTML to be rendered rather than a page at some Uri. The example creates a simple html button which uses window.external.notify to pass a message to the WebBroswer control via it's ScriptNotify event.
XAML:
<Grid x:Name="LayoutRoot" Background="White">
<WebBrowser Name="webBrowser"
ScriptNotify="webBrowser_ScriptNotify">
</WebBrowser>
</Grid>
Code Behind:
using System.Windows.Controls;
using System.Text;
using System.Windows;
namespace SL4HTMLHosting
{
public partial class MainPage : UserControl
{
public MainPage()
{
InitializeComponent();
// Create some basic HTML on the fly containing a simple button,
// that when clicked passes a message to the containing WebBrowser
var html = new StringBuilder();
html.Append("<html>");
html.Append(" <body>");
html.Append(@" <input type=""button"" value=""Show Message Box"" onclick=""window.external.notify('button click');"" /> ");
html.Append(" </body>");
html.Append("</html>");
webBrowser.NavigateToString(html.ToString());
}
private void webBrowser_ScriptNotify(object sender, NotifyEventArgs e)
{
MessageBox.Show(string.Format("Message from web page: '{0}'.",e.Value));
}
}
}
SHARE: