In a previous post I wrote about Customising the Appearance of Debug Information in Visual Studio with the DebuggerDisplay Attribute. In addition to controlling the high level debugger appearance of an object we can also exert a lot more control over how the object appears in the debugger by using the DebuggerTypeProxy attribute.
For example, suppose we have the following (somewhat arbitrary) class:
class DataTransfer
{
public string Name { get; set; }
public string ValueInHex { get; set; }
}
By default, in the debugger it would look like the following:
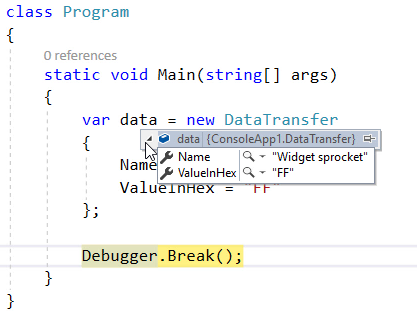
To customize the display of the object members, the DebuggerTypeProxy attribute can be applied.
The first step is to create a class to act as a display proxy. This class takes the original object as part of the constructor and then exposes the custom view via public properties.
For example, suppose that we wanted a decimal display of the hex number that originally is stored in a string property in the original DataTransfer object:
class DataTransferDebugView
{
private readonly DataTransfer _data;
public DataTransferDebugView(DataTransfer data)
{
_data = data;
}
public string NameUpper => _data.Name.ToUpperInvariant();
public string ValueDecimal
{
get
{
bool isValidHex = int.TryParse(_data.ValueInHex, System.Globalization.NumberStyles.HexNumber, null, out var value);
if (isValidHex)
{
return value.ToString();
}
return "INVALID HEX STRING";
}
}
}
Once this view object is defined, it can be selected by decorating the DataTransfer class with the DebuggerTypeProxy attribute as follows:
[DebuggerTypeProxy(typeof(DataTransferDebugView))]
class DataTransfer
{
public string Name { get; set; }
public string ValueInHex { get; set; }
}
Now in the debugger, the following can be seen:
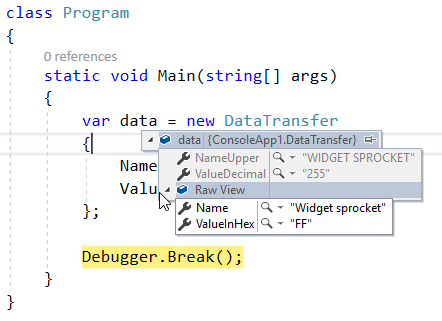
Also notice in the preceding image, that the original object view is available by expanding the Raw View section.
To learn more about C# attributes and even how to create your own custom ones, check out my C# Attributes: Power and Flexibility for Your Code Pluralsight course.You can start watching with a Pluralsight free trial.


SHARE: