Silverlight 4 added the static Clipboard class with the clearly-named SetText, GetText and ContainsText methods.
If running in-browser or out-of-browser without elevated trust the Clipboard methods can only be called as a result of user actions (such as Click, KeyDown). The first time a method is called, a security prompt is presented to the user asking them to grant the app access to the clipboard.
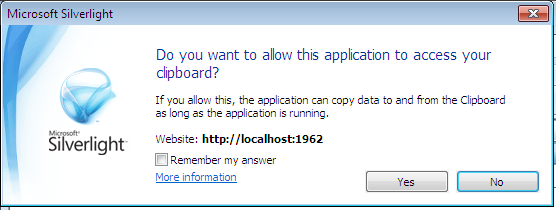
If the user selects 'No' a SecurityException is thrown.
If the app is running out-of-browser with elevated trust, the above dialog is not shown and the Clipboard methods can be called even if not in response to user initiated events such as in your page constructor, in a DispatcherTimer.Tick event, etc.
Example Code
<Button x:Name="btnCopy" Click="btnCopy_Click">Copy</Button>
<Button x:Name="btnPaste" Click="btnPaste_Click">Paste</Button>
<TextBox x:Name="txtBox"></TextBox>
private void btnCopy_Click(object sender, RoutedEventArgs e)
{
// Assumes we want to prevent the user from copy 'nothing' to the clipboard.
// Without this check, whatever text is currently in the clipboard will be replaced
// with nothing.
if (!string.IsNullOrEmpty(txtBox.Text))
{
try
{
Clipboard.SetText(txtBox.Text);
}
catch (SecurityException ex)
{
// If the user does not grant access for the app to access the clipboard
// a SecurityException is thrown
MessageBox.Show("Clipboard access is required to use this feature.");
}
}
}
private void btnPaste_Click(object sender, RoutedEventArgs e)
{
try
{
txtBox.Text = Clipboard.GetText();
}
catch (SecurityException ex)
{
// If the user does not grant access for the app to access the clipboard
// a SecurityException is thrown
MessageBox.Show("Clipboard access is required to use this feature.");
}
}
SHARE: