SQLite is a popular embedded database in use in Windows Store and Windows Phone apps, thought it can sometimes be tricky to get setup: there are a few different choices of NuGet packages / Visual Studio extensions to get up and running with.
An alternative embeddable database to use in Windows Store apps is BrightstarDB.
BrightstarDB is embeddable like SQLite but one big difference is that it’s a NoSQL database based on RDF. It still remains accessible to .NET developers via its entity framework like layer that supports niceties such as LINQ. BrightstarDB can also be used in desktop/web applications in addition to Windows Phone (Silverlight) apps.
Usage
First, in a Windows Store project install the NuGet packages: “BrightstarDB” and “BrightstarDB.Platform”
This will install the relevant libraries and will also create a file called “MyEntityContext.tt” which will generate a strongly typed data context for us to work with.
To define an entity, an interface is used and marked with the [Entity] attribute:
[Entity]
interface IToDoItem
{
string Id { get; }
string Description { get; set; }
}
Now when the MyEntityContext.tt file is right clicked and the option Run Custom Tool is selected, the MyEntityContext.cs file will be generated that knows how to save and retrieve ToDoItems. Entities can also be defined with relationships.
As a basic user interface that allows creation of a to do and loading existing todos in alphabetical order:
<Page
x:Class="ToDo.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}" Width="300">
<StackPanel>
<TextBox PlaceholderText="New task description" Name="NewDescription"></TextBox>
<Button Name="Create" Click ="Create_OnClick">Create</Button>
<Button Name="Load" Click ="Load_OnClick">Load</Button>
<ListView Name="Tasks" DisplayMemberPath="Description"></ListView>
</StackPanel>
</Grid>
</Page>
To implement the code that saves a new ToDoItem to the embedded database:
private const string _connectionString = "type=embedded;storesDirectory=data;storename=tasksdb";
private void Create_OnClick(object sender, RoutedEventArgs e)
{
using (var ctx = new MyEntityContext(_connectionString))
{
var newTask = ctx.ToDoItems.Create();
newTask.Description = NewDescription.Text;
ctx.SaveChanges();
}
}
This click handler creates an instance of the generated MyEntityContext, creates a new entity, sets some property values, and finally calls SaveChanges() to write the changes to the underlying database store. Also note the BrightstarDB connection string field.
To retrieve data:
private void Load_OnClick(object sender, RoutedEventArgs e)
{
using (var ctx = new MyEntityContext(_connectionString))
{
Tasks.ItemsSource = ctx.ToDoItems.OrderBy(x => x.Description);
}
}
Here we’re using some LINQ to sort by description and assign the result to the list in the UI.
So if we add the values in the order “Zulu”, “Tango”, “Foxtrot”; when we retrieve them they will look like the following screenshot:
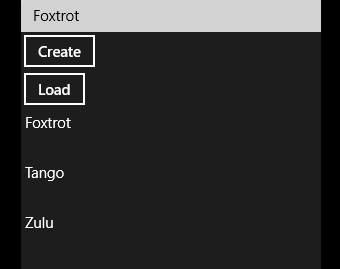
For more information check out the documentation or my Introduction to BrightstarDB Pluralsight Course.
SHARE: