Although code snippets can make creating our initial viewmodels easier (properties, commands,etc.) it’s still tantamount to boilerplate code. Obviously the actions that happen when a command is executed is not boilerplate, but the actual definition is.
T4 templates are built into Visual Studio and allow us to define templates that are a mix of literal output (such as HTML tags) and C# code.
We can for example create a C# for loop around some literal output, e.g. “Hello World” to output it 10 times. For more info on T4, check out MSDN.
T4 for View Model Code Generation
Rather than hand-coding viewmodels, we can define a T4 template. In this template we are able to define the view models we want generating – included both commands and properties that we want to have on those view models.
The implementation in this article creates a new abstract base class for each view model that contains our commands and properties, in addition to a command init method to create and wire-up new MVVM Light RelayCommands along with can execute hooks.
Once T4 has generated these base class view models, we inherit from them and a constructor that calls the base class’s InitCommands method.
The sample application bind some text and a button:
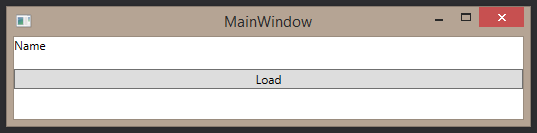
When you click the button the bound Person in the viewmodel is updated and the command is disabled:
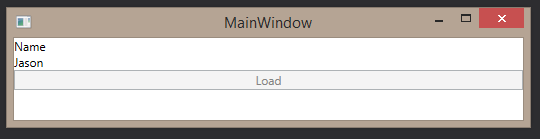
The actual viewmodel code looks like this:
using SampleWpfApplication.Model;
namespace SampleWpfApplication.ViewModel
{
class MainViewModel : MainViewModelBase
{
public MainViewModel()
{
InitCommands();
}
protected override void ExecuteLoad()
{
Who = new Person {Name="Jason"};
LoadCommand.RaiseCanExecuteChanged();
}
protected override bool CanExecuteLoad()
{
return Who == null;
}
}
}
Note it derives from MainViewModelBase which is the generated code.
Also note there’s no Person property (called “Who”) and no RelayCommand defined.
We’ve overridden a couple of methods from the base class to get the behaviour we want and also called InitCommands in the constructor.
The fragment of the T4 template that defines the viewmodels looks like this:
/// <summary>
/// Make changes to this class to define what view models you want generating
/// </summary>
public static class ViewModelGeneratorSettings
{
// ********** Which name space the view model classes will live in
public const string OutputNameSpace = "SampleWpfApplication.ViewModel";
public static List<ViewModelDefinition> ViewModelDefinitions
{
get
{
return new List<ViewModelDefinition>()
{
// Define your view models here
new ViewModelDefinition
{
Name = "MainViewModel",
Properties =
{
Tuple.Create("Who", "Person"), // here Who is the name of the command prop, Person is the type
//Tuple.Create("NextTopic", "Topic"),
//Tuple.Create("CurrentTopicTimeRemaining", "TimeSpan"),
//Tuple.Create("TotalTimeRemaining", "TimeSpan"),
//Tuple.Create("IsPlaying", "bool") // here IsPlaying is the name of the command prop, bool is the type
},
Commands =
{
"Load", // Name of the commands
//"Pause"
}
},
new ViewModelDefinition
{
Name = "AnotherViewModel",
Properties =
{
Tuple.Create("SomeProperty", "int"),
},
Commands =
{
"A",
"B"
}
}, // etc.
};
}
}
}
To create new viewmodels we just add new ViewModelDefinitions and specify what properties and commands we want – currently property types are specified as strings rather than actual types.
To get started and see it in action, download the sample application from GitHub and see how it fits together. The full template is here as well.
While the examples here relate to MVVM Light, the concept could be used with other frameworks.
SHARE: