When we need to generate a sequence of integer values, we can do it manually using some kind of loop, or we make use of the Enumerable.Range method.
The following code illustrates:
var oneToTen = Enumerable.Range(1, 10);
int[] twentyToThirty = Enumerable.Range(20, 11).ToArray();
List<int> oneHundredToOneThirty = Enumerable.Range(100, 31).ToList();
We can also use the results of .Range and transform them in some way, for example to get the letters of the alphabet we could write something like this:
var alphabet = Enumerable.Range(0, 26).Select(i => Convert.ToChar('A' + i));
This will generate an IEnumerable<char> containing the letters A through Z.
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE:
The equality performance of struct equality comparisons can be improved by overriding .Equals(). This is especially true if the structs we are comparing contain reference type fields.
By default, the equality of structs is automatically determined by doing a byte-by-byte comparison of the two struct objects in memory – only when the structs don’t contain any reference types. When the structs contain reference type fields then reflection is used to compare the fields of the two struct objects which results in slower performance.
This chart show the relative performance of the default equality of a struct that contains only value types against a struct that also contains a reference type:
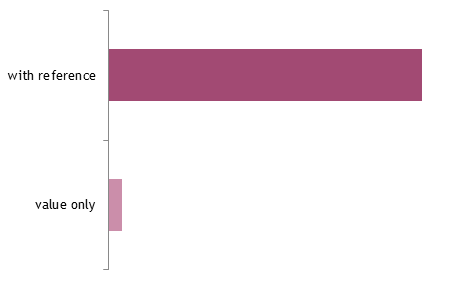
More...
SHARE:
We can create generic classes such as:
class ThingWriter<T>
{
public void Write(T thing)
{
Console.WriteLine(thing);
}
}
Here we’re defining a generic class, then using the type T as a method parameter in the Write method. To use this class we’d write something like:
var w = new ThingWriter<int>();
w.Write(42);
We don’t however have to be working in a generic class to make use of generic methods.
More...
SHARE:
If we’ve got chars with numeric digits in them, we can convert them to numeric (double) values using the char.GetNumericValue method.
The following code:
double d = char.GetNumericValue('5');
Console.WriteLine(d);
Outputs the value: 5
So why does GetNumericValue return a double when a char can only be a single character and hold a single “number” (‘0’ to ‘9’)? Because char holds Unicode characters.
So the following code:
double d = char.GetNumericValue('⅔');
Console.WriteLine(d);
Outputs the value: 0.666666666666667
Hence the need for GetNumericValue to return a double.
For more C# tips check out my Pluralsight courses: C# Tips and Traps and C# Tips and Traps 2.
You can start watching with a Pluralsight free trial.


SHARE:
My previous article “Non Short Circuiting C# Conditional Operators” garnered some comments which suggested to me that I should expand on it. There can sometimes be some confusion over the & and && operator in C# so hopefully this article goes some way to helping clarify the differences between them.
The && Operator
Works with: bools
The && operator performs a logical AND on Boolean values.
For example, the following code:
bool b1 = true;
bool b2 = false;
bool isBothTrue = b1 && b2;
Console.WriteLine(isBothTrue);
Outputs:
False
If we get our two bool values from the result of a couple of methods instead, and the first method returns false, the && operator won’t execute the second method. The following code:
More...
SHARE:
Most of the time when writing C# programs we use the short-circuiting conditional operators. These operators do not continue evaluation once the outcome has been determined.
Take this code:
bool b = false && CheckName(name);
b = false & CheckName(name);
Here we’re performing a logical AND with the value false and the result of the CheckName method. Because we have a false, the logical AND can never be true.
The first statement using the short-circuiting AND operator (“&&”) will not execute the CheckName method because as soon as it’s determined the outcome (the first value is false and we are AND-ing) the remaining terms are not even evaluated.
More...
SHARE:
My latest Pluralsight course has just been released. It’s the follow-up course to the popular first C# Tips and Traps course.
Course Description
Whether you're still learning C# or you already have some experience, it's sometimes hard to know what you don't know. This is the follow-up course to C# Tips and Traps and is designed to further short-circuit your C# learning and provides a whole host of useful information about the sometimes under-used or unknown features of both the C# language and the .Net framework. It's suitable for those who are brand new to C# as well as experienced developers looking to "round off" their C# skills and "fill in the gaps".
Check out the course table of contents for more details.
You can start watching with a Pluralsight free trial.


SHARE:
It’s possible to use C# keywords for variable names, etc.
For example if we wanted a variable called “namespace” (which is a C# keyword) we can prefix the declaration with an @ and use this prefix whenever we refer to the variable:
var @namespace = "hello";
@namespace += " world";
More...
SHARE:
When we’re formatting numbers we can use a format string that allows us to specify a different format to be used whenever the number is positive, negative or zero.
To do this we separate the 3 parts by a semicolon.
For example the format string "(+)#.##;(-)#.##;(sorry nothing at all)" will output:
- (+)99.99 if the value was 99.99
- (-)23.55 if the value was -23.55
- (sorry nothing at all) if the value was zero
You can also use a two part conditional format string where the first part will be used if the number is positive or zero; the second part will be used if the number is negative.
Check out the MSDN page for more info…
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE:
If we’re copying arrays where the types may be different, sometimes we may want to do an atomic copy; that is if any of the elements in the source array can’t be converted to the destination array type, the whole copy operation will “rollback” and the target array will remain unchanged.
The Array.ConstrainedCopy method can do this for us.
Consider the code below where an array of objects (containing a string and and an int) is copied to a target array of type string.
Using .CopyTo will result in an exception and the target array being partially affected (the target array element 0 will have already been populated with the string).
Using .ConstrainedCopy will ensure that if an exception occurs the target array will be “rolled back”, i.e. none of it’s elements will have been changed.
var things = new object[] { "Sarah", 1};
var strings = new string[2];
// this will throw an exception because of the 2nd element conversion error (int to string)
// but, the target array "strings" would have been changed (the 1st string element will have been copied)
things.CopyTo(strings, 0);
// this will throw an exception because of the 2nd element conversion error (int to string)
// BUT, the target array "strings" will NOT have been changed
Array.ConstrainedCopy(things, 0, strings, 0, 2);
The constrained copy has the following parameters (from MSDN):
public static void ConstrainedCopy(
Array sourceArray,
int sourceIndex,
Array destinationArray,
int destinationIndex,
int length
)
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE: