The Console class can do more than just WriteLine().
Here’s 3 fun/weird/useful/annoying things.
1. Setting The Console Window Size
The Console.SetWindowSize(numberColumns, numberRows) method sets the console window size.
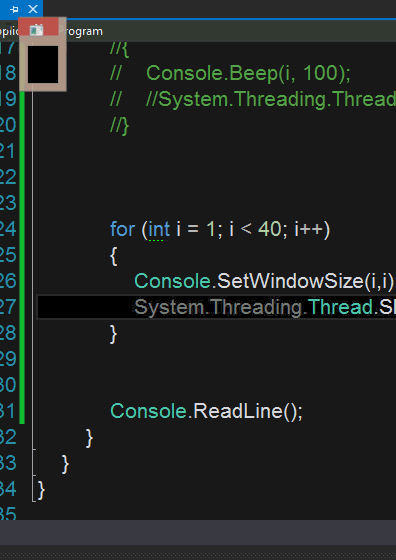
To annoy your users (or create a “nice” console opening animation) as this animated GIF shows you could write something like:
for (int i = 1; i < 40; i++)
{
Console.SetWindowSize(i,i);
System.Threading.Thread.Sleep(50);
}
2. Beeping Consoles
The Console.Beep() method emits a beep from the speaker(s).
We can also specify a frequency and duration.
More...
SHARE:
The Zip method allows us to join IEnumerable sequences together by interleaving the elements in the sequences.
Zip is an extension method on IEnumerable. For example to zip together a collection of names with ages we could write:
var names = new [] {"John", "Sarah", "Amrit"};
var ages = new[] {22, 58, 36};
var namesAndAges = names.Zip(ages, (name, age) => name + " " + age);
This would produce an IEnumerable<string> containing three elements:
- “John 22”
- “Sarah 58”
- “Amrit 36”
If one sequence is shorter that the other, the “zipping” will stop when the end of the shorter sequence is reached. So if we added an extra name:
var names = new [] {"John", "Sarah", "Amrit", "Bob"};
We’d end up with the same result as before, “Bob” wouldn’t be used as there isn’t a corresponding age for him.
We can also create objects in our lambda, this example shows how to create an IEnumerable of two-element Tuples:
var names = new [] {"John", "Sarah", "Amrit"};
var ages = new[] {22, 58, 36};
var namesAndAges = names.Zip(ages, (name, age) => Tuple.Create(name, age));
This will produce an IEnumerable<Tuple<String,Int32>> that contains three Tuples, with each Tuple holding a name and age.
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE:
When we need to generate a sequence of integer values, we can do it manually using some kind of loop, or we make use of the Enumerable.Range method.
The following code illustrates:
var oneToTen = Enumerable.Range(1, 10);
int[] twentyToThirty = Enumerable.Range(20, 11).ToArray();
List<int> oneHundredToOneThirty = Enumerable.Range(100, 31).ToList();
We can also use the results of .Range and transform them in some way, for example to get the letters of the alphabet we could write something like this:
var alphabet = Enumerable.Range(0, 26).Select(i => Convert.ToChar('A' + i));
This will generate an IEnumerable<char> containing the letters A through Z.
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE:
xUnit.net allows the creation of data-driven tests. These kind of tests get their test data from outside the test method code via parameters added to the test method signature.
Say we had to test a Calculator class and check it’s add method returned the correct results for six different test cases. Without data-driven tests we’d either have to write six separates tests (with almost identical code) or some loop inside our test method containing an assert.
Regular xUnit.net test methods are identified by applying the [Fact] attribute. Data-driven tests instead use the [Theory] attribute.
To get data-driven features and the [Theory] attribute, install the xUnit.net Extensions NuGet package in addition to the standard xUnit.net package.
Creating Inline Data-Driven Tests
The [InlineData] attribute allows us to specify test data that gets passed to the parameters of test method.
So for our Calculator add test we’d start by defining the test method:
More...
SHARE:
The equality performance of struct equality comparisons can be improved by overriding .Equals(). This is especially true if the structs we are comparing contain reference type fields.
By default, the equality of structs is automatically determined by doing a byte-by-byte comparison of the two struct objects in memory – only when the structs don’t contain any reference types. When the structs contain reference type fields then reflection is used to compare the fields of the two struct objects which results in slower performance.
This chart show the relative performance of the default equality of a struct that contains only value types against a struct that also contains a reference type:
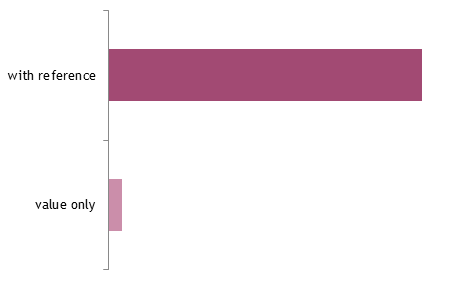
More...
SHARE:
To help people get started with xUnit.net and as an accompaniment to my Pluralsight xUnit.net training course I thought I’d create a cheat sheet showing common assert methods and attributes. Hopefully will be of use :)
More...
SHARE:
My latest Pluralsight course on the xUnit.net testing framework has just been released.
Course Description
Learn the latest in unit testing technology for C#, VB.NET (and other .NET languages) created by the original inventor of NUnit.
xUnit.net is a free, extensible, open source framework designed for programmers that aligns more closely with the .NET platform.
You can check it out now on Pluralsight.com.
You can start watching with a Pluralsight free trial.


SHARE:
We can create generic classes such as:
class ThingWriter<T>
{
public void Write(T thing)
{
Console.WriteLine(thing);
}
}
Here we’re defining a generic class, then using the type T as a method parameter in the Write method. To use this class we’d write something like:
var w = new ThingWriter<int>();
w.Write(42);
We don’t however have to be working in a generic class to make use of generic methods.
More...
SHARE:
If we’ve got chars with numeric digits in them, we can convert them to numeric (double) values using the char.GetNumericValue method.
The following code:
double d = char.GetNumericValue('5');
Console.WriteLine(d);
Outputs the value: 5
So why does GetNumericValue return a double when a char can only be a single character and hold a single “number” (‘0’ to ‘9’)? Because char holds Unicode characters.
So the following code:
double d = char.GetNumericValue('⅔');
Console.WriteLine(d);
Outputs the value: 0.666666666666667
Hence the need for GetNumericValue to return a double.
For more C# tips check out my Pluralsight courses: C# Tips and Traps and C# Tips and Traps 2.
You can start watching with a Pluralsight free trial.


SHARE:
My previous article “Non Short Circuiting C# Conditional Operators” garnered some comments which suggested to me that I should expand on it. There can sometimes be some confusion over the & and && operator in C# so hopefully this article goes some way to helping clarify the differences between them.
The && Operator
Works with: bools
The && operator performs a logical AND on Boolean values.
For example, the following code:
bool b1 = true;
bool b2 = false;
bool isBothTrue = b1 && b2;
Console.WriteLine(isBothTrue);
Outputs:
False
If we get our two bool values from the result of a couple of methods instead, and the first method returns false, the && operator won’t execute the second method. The following code:
More...
SHARE: