Windows Services allow us to run code in the background, without needing (for example) a console application running continually. Windows Services can run as various system users or specific local/domain users.
To see what Windows Services are installed on your (Win 8+) PC, hit Win+x then hit G. In the Computer Management window that opens, click the Services option under Services and Applications as shown in the following screenshot.
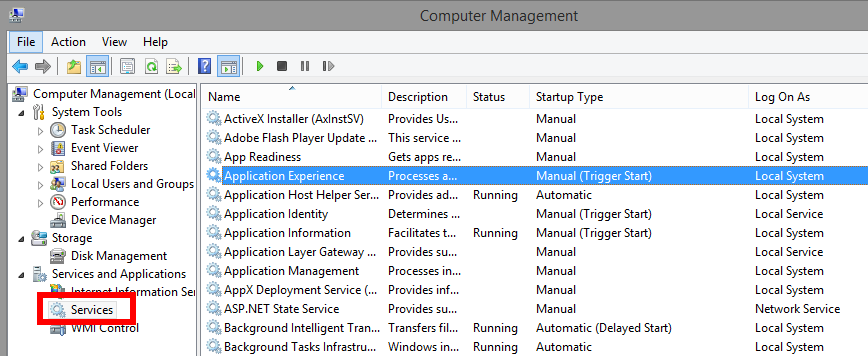
As the preceding screenshot shows, a Service has a (Display) Name, a Description, a Status (e.g. Running or not), a Startup Type (Manual, Automatic, Disabled, etc), and a Log On As that specifies in which user context the Service executes.
We can create Windows Services to run arbitrary .NET code such as:
- Self-hosted web server using Owin
- File system watcher and batch processing (e.g. image file conversion, video encoding)
- Host remote Akka.NET actor system (such as in my Pluralsight course)
- Process messages from a message queue as they arrive (e.g. MSMQ)
- Mail, FTP, etc. servers
- Integration/gateway, e.g. receive data from external systems
Using Topshelf
Topshelf is an open source project that greatly simplifies the creation of Windows Services.
The overview of creating a Service using Topshelf is:
- Create a Console application project in Visual Studio
- Add the Topshelf NuGet package
- Create a class to represent your service logic
- Configure your Service using the Topshelf API
- Build your Console application
- Execute your Console application passing Topshelf parameters to install/uninstall your Service
So assuming we have a new Console project called “Time” and the following NuGet packages are installed:
<packages>
<package id="Microsoft.AspNet.WebApi.Client" version="5.2.3" targetFramework="net451" />
<package id="Microsoft.AspNet.WebApi.Core" version="5.2.3" targetFramework="net451" />
<package id="Microsoft.AspNet.WebApi.Owin" version="5.2.3" targetFramework="net451" />
<package id="Microsoft.AspNet.WebApi.OwinSelfHost" version="5.2.3" targetFramework="net451" />
<package id="Microsoft.Owin" version="2.0.2" targetFramework="net451" />
<package id="Microsoft.Owin.Host.HttpListener" version="2.0.2" targetFramework="net451" />
<package id="Microsoft.Owin.Hosting" version="2.0.2" targetFramework="net451" />
<package id="Newtonsoft.Json" version="6.0.4" targetFramework="net451" />
<package id="Owin" version="1.0" targetFramework="net451" />
<package id="Topshelf" version="3.2.0" targetFramework="net451" />
</packages>
We can create a self-hosted OWIN service that tells us the time.
First off we create an API Controller to return the time and then create an OWIN startup configuration class:
public class TimeController : ApiController
{
[HttpGet]
public string Now()
{
return DateTime.Now.ToLongTimeString();
}
}
public class Startup
{
public void Configuration(IAppBuilder appBuilder)
{
var config = new HttpConfiguration();
config.Routes.MapHttpRoute("DefaultApi", "api/{controller}/{time}");
config.Formatters.Remove(config.Formatters.XmlFormatter);
config.Formatters.Add(config.Formatters.JsonFormatter);
appBuilder.UseWebApi(config);
}
}
Next we create a class that represents our Topshelf Windows Service:
class TimeService
{
private IDisposable _webServer;
public void Start()
{
// code that runs when the Windows Service starts up
_webServer = WebApp.Start<Startup>("http://localhost:8084");
}
public void Stop()
{
// code that runs when the Windows Service stops
_webServer.Dispose();
}
}
Now in the Console applications main method we can use Topshelf’s HostFactory to configure what our Windows Service will do (via the TimeService class) and how it will be configured in Windows:
class Program
{
static void Main(string[] args)
{
HostFactory.Run(
configuration =>
{
configuration.Service<TimeService>(
service =>
{
service.ConstructUsing(x => new TimeService());
service.WhenStarted(x => x.Start());
service.WhenStopped(x => x.Stop());
});
configuration.RunAsLocalSystem();
configuration.SetServiceName("ASimpleService");
configuration.SetDisplayName("A Simple Service");
configuration.SetDescription("Don't Code Tired Demo");
});
}
}
Once the solution is built, navigate to the bin/debug directory in a admin command prompt and type:
time.exe install
This will install the Service into Windows as the following screenshots show.
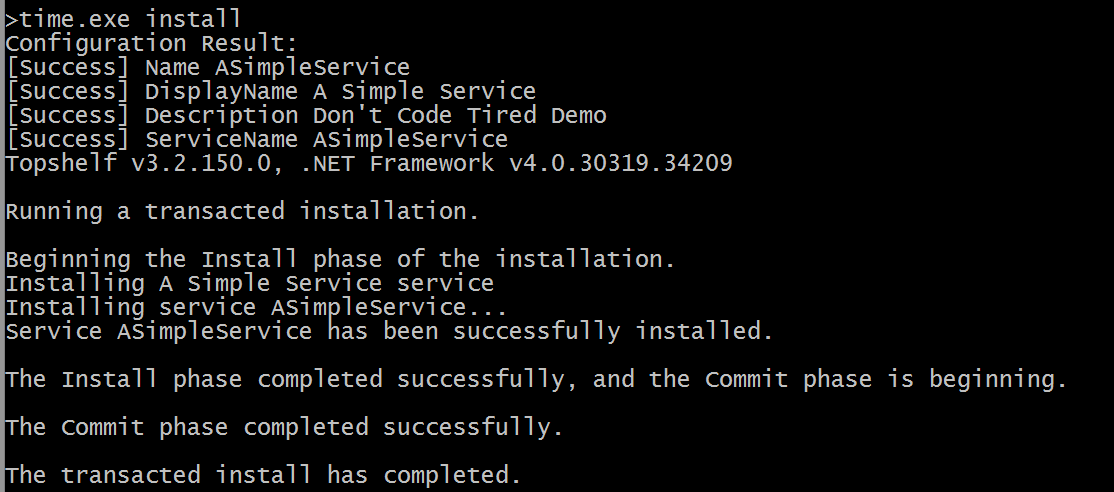
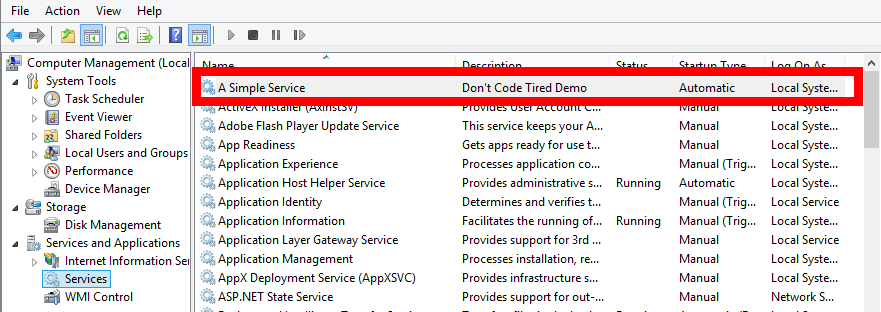
In the command prompt window type:
time.exe start
This will start our new service.
Now in a browser, navigate to http://localhost:8084/api/time/now and you’ll see the current time come back as JSON
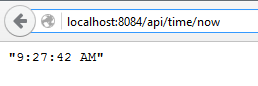
To learn more about Topshelf, check out my Pluralsight course: Getting Started Building Windows Services with Topshelf.
You can start watching with a Pluralsight free trial.


SHARE: